When it comes to automation, there are so many tools available on the market. No matter how many tools are there, one of the biggest problems we face in automation is the maintainability of the script. As you are aware, one of the main issues we face is the continuous changes in the element locators we have to do.
I was thinking about a solution for this exact problem and came out with a handy solution.
First, let’s look at our problem
- There are elements with the same name, id ( this happens due to developer-human errors but we can’t avoid it )
- The CSS class names change time to time
- New divs, paragraphs, tables get integrated into the webpage and it makes it harder to maintain a stable XPath
This list will go on, so when there is a change, we have to capture the XPath, or maybe change the id or change the locator, and so on.
Instead of the above changes, what if you just have to do a small number change? Or what if despite the class, id changes, or new element additions, your script works smoothly.
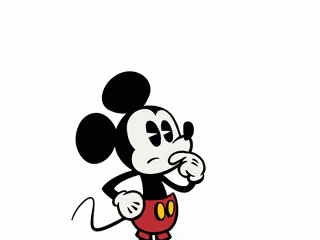
Let’s take the Facebook login page for our example. On the Facebook login page, let’s say we wanted to insert our username and password. If you are a selenium user it will be driver.findelement(By.id(“login).sendkeys… and if you are a cypress user you will be using cy.get(“#login”).type…
In the way that I’m suggesting, we do not go after individual elements. Instead, at the beginning of the test, we make a repository of all the elements we need using their TAGNAME. I originally got this idea via going through the js method getElementsByTagName. If you want to get an idea of what I’m talking about, go to the console of your prefered web browser and user the command document.getElementsByTagName(“input”)
As I said, what we will get is a list of objects (web elements). If you use the word input, you will get all the elements with input tags. Then in the list, you can exactly find out what is the input element which you need to use. The advantage of this is since you are selecting the exact object you need to access to from a list of objects, even though there are similar names and id’s it won’t be a problem to you anymore.
Let me show how this is done using Cypress.
Saying we need to login to Facebook,
We will maintain a JSON file to keep an index mapping to our object list. A sample JSON file will look like this,
Here I have found the index 16 of the inputs list of facebook.com maps the username field and index 17 maps the password field
Same as above, from buttons, index 0 maps with the sign-in button.

Now the Rest is simple,
After you load the page, all you need to do is get all the web element objects and extract the objects you need.

As mentioned in the script above, The bellow command will get all the input objects to inputs variable and you can simply access all the elements using their index ( ex: inputs[16] )
cy.document().then((doc) => {
const inputs = doc.getElementsByTagName(‘input’)})
As I said, once you created the script in need of an element change, the only change that you need to do will be the change in the index in the JSON file we mentioned earlier.
If you are a selenium user, you can use the selenium method driver.findElements(By.tagName(“input”)) to do the same thing. I will add a sample of a Selenium+Testng — Java code too. I just wrote it for demonstration purposes so it’s not properly formatted. But hope you will get the idea
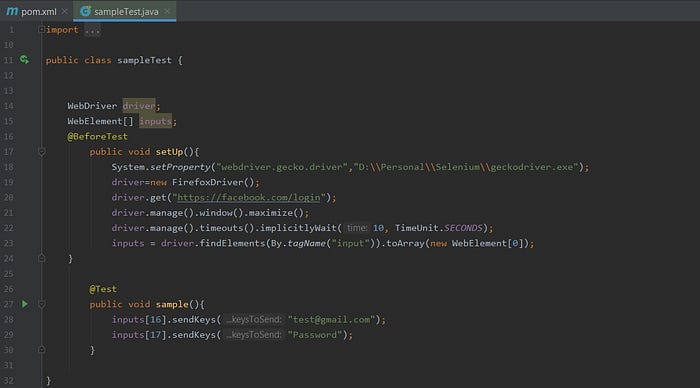
Instead of a JSON file, you can even use a properties file or any other data storing method you prefer.
The best part is, you can just copy-paste some of the scripts for the products with the same behavior and run it with just an index change of the element map ;)
Hope this helps.
Cheers,
Muditha Perera